spawnia / sailor
A typesafe GraphQL client for PHP
Fund package maintenance!
spawnia
Installs: 320 779
Dependents: 2
Suggesters: 0
Security: 0
Stars: 85
Watchers: 7
Forks: 18
Open Issues: 4
Requires
- php: ^7.4 || ^8
- ext-json: *
- nette/php-generator: ^3.6.9 || ^4.1.7
- psr/http-client: ^1
- symfony/console: ^5 || ^6 || ^7
- symfony/var-exporter: ^5.3 || ^6 || ^7
- thecodingmachine/safe: ^1 || ^2 || ^3
- webonyx/graphql-php: ^14.11.3 || ^15
Requires (Dev)
- bensampo/laravel-enum: ^3 || ^4.1 || ^5 || ^6
- composer/composer: ^2
- ergebnis/composer-normalize: ^2.13
- friendsofphp/php-cs-fixer: ~3.63.0
- guzzlehttp/guzzle: ^7
- jangregor/phpstan-prophecy: ^1 || ^2
- mll-lab/php-cs-fixer-config: ^5
- mockery/mockery: ^1.4
- nesbot/carbon: ^2.73 || ^3
- nyholm/psr7: ^1.4
- ocramius/package-versions: ^1 || ^2
- php-http/httplug: ^2
- php-http/mock-client: ^1.4
- phpstan/extension-installer: ^1
- phpstan/phpstan: ^1.12.19 || ^2
- phpstan/phpstan-deprecation-rules: ^1 || ^2
- phpstan/phpstan-mockery: ^1 || ^2
- phpstan/phpstan-phpunit: ^1 || ^2
- phpstan/phpstan-strict-rules: ^1 || ^2
- phpunit/phpunit: ^9.6.22 || ^10.5.45 || ^11.5.10 || ^12.0.5
- spawnia/phpunit-assert-directory: ^2.1
- symfony/var-dumper: ^5.2.3 || ^6 || ^7
- thecodingmachine/phpstan-safe-rule: ^1.1
Suggests
- bensampo/laravel-enum: Use with BenSampoEnumTypeConfig
- guzzlehttp/guzzle: Enables using the built-in default Client
- mockery/mockery: Used in Operation::mock()
- nesbot/carbon: Use with CarbonTypeConfig
- dev-master
- v1.1.1
- v1.1.0
- v1.0.0
- v0.35.0
- v0.34.0
- v0.33.1
- v0.33.0
- v0.32.2
- v0.32.1
- v0.32.0
- v0.31.2
- v0.31.1
- v0.31.0
- v0.30.1
- v0.30.0
- v0.29.3
- v0.29.2
- v0.29.1
- v0.29.0
- v0.28.2
- v0.28.1
- v0.28.0
- v0.27.0
- v0.26.1
- v0.26.0
- v0.25.0
- v0.24.0
- v0.23.1
- v0.23.0
- v0.22.0
- v0.21.2
- v0.21.1
- v0.21.0
- v0.20.2
- v0.20.1
- v0.20.0
- v0.19.0
- v0.18.2
- v0.18.1
- v0.18.0
- v0.17.1
- v0.17.0
- v0.16.0
- v0.15.0
- v0.14.1
- v0.14.0
- v0.13.0
- v0.12.0
- v0.11.0
- v0.10.2
- v0.10.1
- v0.10.0
- v0.9.0
- v0.8.0
- v0.7.0
- v0.6.0
- v0.5.0
- v0.4.2
- v0.4.1
- v0.4.0
- v0.3.0
- v0.2.0
- v0.1.0
- dev-fix-properties-name-conflict
- dev-skip-non-nullable
This package is auto-updated.
Last update: 2025-06-24 06:12:22 UTC
README
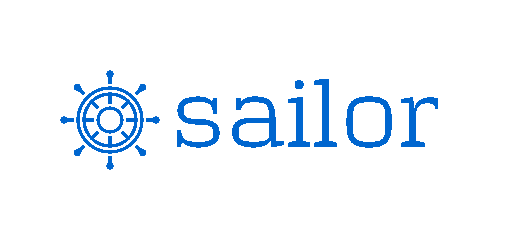
A typesafe GraphQL client for PHP
Motivation
GraphQL provides typesafe API access through the schema definition each server provides through introspection. Sailor leverages that information to enable an ergonomic workflow and reduce type-related bugs in your code.
The native GraphQL query language is the most universally used tool to formulate GraphQL queries and works natively with the entire ecosystem of GraphQL tools. Sailor takes the plain queries you write and generates executable PHP code, using the server schema to generate typesafe operations and results.
Installation
Install Sailor through composer by running:
composer require spawnia/sailor
If you want to use the built-in default Client (see Client implementations):
composer require guzzlehttp/guzzle
If you want to use the PSR-18 Client and don't have PSR-17 Request and Stream factory implementations (see Client implementations):
composer require nyholm/psr7
Configuration
Run vendor/bin/sailor
to set up the configuration.
A file called sailor.php
will be created in your project root.
A Sailor configuration file is expected to return an associative array
where the keys are endpoint names and the values are instances of Spawnia\Sailor\EndpointConfig
.
You can take a look at the example configuration to see what options
are available for configuration: sailor.php
.
If you would like to use multiple configuration files,
specify which file to use through the -c/--config
option.
It is quite useful to include dynamic values in your configuration.
You might use PHP dotenv to load
environment variables (run composer require vlucas/phpdotenv
if you do not have it installed already.).
# sailor.php +$dotenv = Dotenv\Dotenv::createImmutable(__DIR__); +$dotenv->load(); ... public function makeClient(): Client { return new \Spawnia\Sailor\Client\Guzzle( - 'https://hardcoded.url', + getenv('EXAMPLE_API_URL'), [ 'headers' => [ - 'Authorization' => 'hardcoded-api-token', + 'Authorization' => getenv('EXAMPLE_API_TOKEN'), ], ] ); }
Client implementations
Sailor provides a few built-in clients:
Spawnia\Sailor\Client\Guzzle
: Default HTTP clientSpawnia\Sailor\Client\Psr18
: PSR-18 HTTP clientSpawnia\Sailor\Client\Log
: Used for testing
You can bring your own by implementing the interface Spawnia\Sailor\Client
.
Dynamic clients
You can configure clients dynamically for specific operations or per request:
use Example\Api\Operations\HelloSailor; /** @var \Spawnia\Sailor\Client $client Somehow instantiated dynamically */ HelloSailor::setClient($client); // Will use $client over the client from EndpointConfig $result = HelloSailor::execute(); // Reverts to using the client from EndpointConfig HelloSailor::setClient(null);
Custom types
Custom scalars are commonly serialized as strings, but may also use other representations.
Without knowing about the contents of the type, Sailor can not do any conversions or provide more accurate type hints, so it uses mixed
.
Since enums are only supported from PHP 8.1 and this library still supports PHP 7.4,
it generates enums as a class with string constants and handles values as string
.
You may leverage native PHP enums by overriding EndpointConfig::enumTypeConfig()
and return an instance of Spawnia\Sailor\Type\NativeEnumTypeConfig
.
Overwrite EndpointConfig::configureTypes()
to specialize how Sailor deals with the types within your schema.
See examples/custom-types.
Error conversion
Errors sent within the GraphQL response must follow the response errors specification.
Sailor converts the plain stdClass
obtained from decoding the JSON response into
instances of \Spawnia\Sailor\Error\Error
by default.
If one of your endpoints returns structured data in extensions
, you can customize how
the plain errors are decoded into class instances by overwriting EndpointConfig::parseError()
.
Usage
Introspection
Run vendor/bin/sailor introspect
to update your schema with the latest changes
from the server by running an introspection query. As an example, a very simple
server might result in the following file being placed in your project:
# schema.graphql type Query { hello(name: String): String }
Define operations
Put your queries and mutations into .graphql
files and place them anywhere within your
configured project directory. You are free to name and structure the files in any way.
Let's query the example schema from above:
# src/example.graphql query HelloSailor { hello(name: "Sailor") }
You must give all your operations unique PascalCase
names, the following example is invalid:
# Invalid, operations have to be named query { anonymous } # Invalid, names must be unique across all operations query Foo { ... } mutation Foo { ... } # Invalid, names must be PascalCase query camelCase { ... }
Generate code
Run vendor/bin/sailor
to generate PHP code for your operations.
For the example above, Sailor will generate a class called HelloSailor
,
place it in the configured namespace and write it to the configured location.
namespace Example\Api\Operations; class HelloSailor extends \Spawnia\Sailor\Operation { ... }
There are additional generated classes that represent the results of calling the operations. The plain data from the server is wrapped up and contained within those value classes, so you can access them in a typesafe way.
Execute queries
You are now set up to run a query against the server,
just call the execute()
function of the new query class:
$result = \Example\Api\Operations\HelloSailor::execute();
The returned $result
is going to be a class that extends \Spawnia\Sailor\Result
and
holds the decoded response returned from the server.
You can just grab the $data
, $errors
or $extensions
off of it:
$result->data // `null` or a generated subclass of `\Spawnia\Sailor\ObjectLike` $result->errors // `null` or a list of `\Spawnia\Sailor\Error\Error` $result->extensions // `null` or an arbitrary map
Error handling
You can ensure an operation returned the proper data and contained no errors:
$errorFreeResult = \Example\Api\Operations\HelloSailor::execute() ->errorFree(); // Throws if there are errors or returns an error free result
The $errorFreeResult
is going to be a class that extends \Spawnia\Sailor\ErrorFreeResult
.
Given it can only be obtained by going through validation,
it is guaranteed to have non-null $data
and does not have $errors
:
$errorFreeResult->data // a generated subclass of `\Spawnia\Sailor\ObjectLike` $errorFreeResult->extensions // `null` or an arbitrary map
If you do not need to access the data and just want to ensure a mutation was successful, the following is more efficient as it does not instantiate a new object:
\Example\Api\Operations\SomeMutation::execute() ->assertErrorFree(); // Throws if there are errors
Queries with arguments
Your generated operation classes will be annotated with the arguments your query defines.
class HelloSailor extends \Spawnia\Sailor\Operation { public static function execute(string $required, ?\Example\Api\Types\SomeInput $input = null): HelloSailor\HelloSailorResult { ... } }
Inputs can be built up incrementally:
$input = new \Example\Api\Types\SomeInput; $input->foo = 'bar';
If you are using PHP 8, instantiation with named arguments can be quite useful to ensure your input is completely filled:
\Example\Api\Types\SomeInput::make(foo: 'bar')
Partial inputs
GraphQL often uses a pattern of partial inputs - the equivalent of an HTTP PATCH
.
Consider the following input:
input SomeInput { requiredID: Int! firstOptional: Int secondOptional: Int }
Suppose we allow instantiation in PHP with the following implementation:
class SomeInput extends \Spawnia\Sailor\ObjectLike { public static function make( int $requiredID, ?int $firstOptional = null, ?int $secondOptional = null, ): self { $instance = new self; $instance->requiredID = $required; $instance->firstOptional = $firstOptional; $instance->secondOptional = $secondOptional; return $instance; } }
Given that implementation, the following call will produce the following JSON payload:
SomeInput::make(requiredID: 1, secondOptional: 2);
{ "requiredID": 1, "firstOptional": null, "secondOptional": 2 }
However, we would like to produce the following JSON payload:
{ "requiredID": 1, "secondOptional": 2 }
This is because from within make()
, there is no way to differentiate between an explicitly
passed optional named argument and one that has been assigned the default value.
Thus, the resulting JSON payload will unintentionally modify firstOptional
too,
erasing whatever value it previously held.
A naive solution to this would be to filter out any argument that is null
.
However, we would also like to be able to explicitly set the first optional value to null
.
The following call should result in a JSON payload that contains "firstOptional": null
.
SomeInput::make(requiredID: 1, firstOptional: null, secondOptional: 2);
In order to generate partial inputs by default, optional named arguments have a special default value:
Spawnia\Sailor\ObjectLike::UNDEFINED = 'Special default value that allows Sailor to differentiate between explicitly passing null and not passing a value at all.';
class SomeInput extends \Spawnia\Sailor\ObjectLike { /** * @param int $requiredID * @param int|null $firstOptional * @param int|null $secondOptional */ public static function make( $requiredID, $firstOptional = 'Special default value that allows Sailor to differentiate between explicitly passing null and not passing a value at all.', $secondOptional = 'Special default value that allows Sailor to differentiate between explicitly passing null and not passing a value at all.', ): self { $instance = new self; if ($requiredID !== self::UNDEFINED) { $instance->requiredID = $requiredID; } if ($firstOptional !== self::UNDEFINED) { $instance->firstOptional = $firstOptional; } if ($secondOptional !== self::UNDEFINED) { $instance->secondOptional = $secondOptional; } return $instance; } }
You may use Spawnia\Sailor\ObjectLike::UNDEFINED
to omit nullable arguments completely:
SomeInput::make( requiredID: 1, firstOptional: $maybeNull ?? Spawnia\Sailor\ObjectLike::UNDEFINED, );
If $maybeNull
is null
, this will result in the following JSON payload:
{ "requiredID": 1 }
In the very unlikely case where you need to pass exactly the value of Spawnia\Sailor\ObjectLike::UNDEFINED
,
you can bypass the logic in make()
and assign it directly:
$input = SomeInput::make(requiredID: 1); $input->secondOptional = Spawnia\Sailor\ObjectLike::UNDEFINED;
Events
Sailor calls EndpointConfig::handleEvent()
with the following events during the execution lifecycle:
- StartRequest: Fired after calling
execute()
on anOperation
, before invoking the client. - ReceiveResponse: Fired after receiving a GraphQL response from the client.
PHP keyword collisions
Since GraphQL uses a different set of reserved keywords, names of fields or types may collide with PHP keywords.
Sailor prevents illegal usages of those names in generated code by prefixing them with a single underscore _
.
Testing
Sailor provides first class support for testing by allowing you to mock operations.
Setup
It is assumed you are using PHPUnit and Mockery.
composer require --dev phpunit/phpunit mockery/mockery
Make sure your test class - or one of its parents - uses the following traits:
use Mockery\Adapter\Phpunit\MockeryPHPUnitIntegration; use PHPUnit\Framework\TestCase as PHPUnitTestCase; use Spawnia\Sailor\Testing\RequiresSailorMocks; abstract class TestCase extends PHPUnitTestCase { use MockeryPHPUnitIntegration; // Makes Mockery assertions work use RequiresSailorMocks; // Prevents stray requests and resets mocks between tests }
If you want to perform some kind of integration test where mocks are not required,
you may replace RequiresSailorMocks
with UsesSailorMocks
.
Mock results
Mocks are registered per operation class:
use Example\Api\Operations\HelloSailor; /** @var \Mockery\MockInterface&HelloSailor */ $mock = HelloSailor::mock();
When registered, the mock captures all calls to HelloSailor::execute()
.
Use it to build up expectations for what calls it should receive and mock returned results:
$name = 'Sailor'; $hello = "Hello, {$name}!"; $mock ->expects('execute') ->once() ->with($name) ->andReturn(HelloSailor\HelloSailorResult::fromData( data: HelloSailor\HelloSailor::make( hello: $hello, ), )); $result = HelloSailor::execute(name: $name) ->errorFree(); self::assertSame($hello, $result->data->hello);
Subsequent calls to ::mock()
will return the initially registered mock instance.
$mock1 = HelloSailor::mock(); $mock2 = HelloSailor::mock(); assert($mock1 === $mock2); // true
You can also simulate a result with errors:
HelloSailor\HelloSailorResult::fromErrors([ (object) [ 'message' => 'Something went wrong', ], ]);
For PHP 8 users, it is recommended to use named arguments to build complex mocked results:
HelloSailor\HelloSailorResult::fromData( data: HelloSailor\HelloSailor::make( hello: 'Hello, Sailor!', nested: HelloSailor\HelloSailor\Nested::make( hello: 'Hello again!', ), ), ))
Integration
If you want to perform integration testing for a service that uses Sailor without actually
hitting an external API, you can swap out your client with the Log
client.
It writes all requests made through Sailor to a file of your choice.
The
Log
client can not know what constitutes a valid response for a given request, so it always responds with an error.
# sailor.php public function makeClient(): Client { return new \Spawnia\Sailor\Client\Log(__DIR__ . '/sailor-requests.log'); }
Each request goes on a new line and contains a JSON string that holds the query
and variables
:
{"query":"{ foo }","variables":{"bar":42}} {"query":"mutation { baz }","variables":null}
This allows you to perform assertions on the calls that were made.
The Log
client offers a convenient method of reading the requests as structured data:
$log = new \Spawnia\Sailor\Client\Log(__DIR__ . '/sailor-requests.log'); foreach ($log->requests() as $request) { var_dump($request); } array(2) { ["query"]=> string(7) "{ foo }" ["variables"]=> array(1) { ["bar"]=> int(42) } } array(2) { ["query"]=> string(7) "mutation { baz }" ["variables"]=> NULL }
To clean up the log after performing tests, use Log::clear()
.
Examples
You can find examples of how a project would use Sailor within examples.
Changelog
See CHANGELOG.md
.
Contributing
See CONTRIBUTING.md
.
License
This package is licensed using the MIT License.