syropian / laravel-notification-channel-throttling
Throttle notifications on a per-channel basis
Installs: 1 772
Dependents: 0
Suggesters: 0
Security: 0
Stars: 9
Watchers: 2
Forks: 0
Open Issues: 1
Requires
- php: ^8.1
- illuminate/mail: ^10.0|^11.0
- illuminate/notifications: ^10.0|^11.0
- illuminate/support: ^10.0|^11.0
- spatie/laravel-package-tools: ^1.14.0
Requires (Dev)
- larastan/larastan: ^2.9
- laravel/pint: ^1.0
- nunomaduro/collision: ^7.8|^8.1
- orchestra/testbench: ^8.8|^9.0
- pestphp/pest: ^2.20
- pestphp/pest-plugin-arch: ^2.0
- pestphp/pest-plugin-laravel: ^2.0
- phpstan/extension-installer: ^1.1
- phpstan/phpstan-deprecation-rules: ^1.0
- phpstan/phpstan-phpunit: ^1.0
This package is auto-updated.
Last update: 2025-06-08 19:37:14 UTC
README
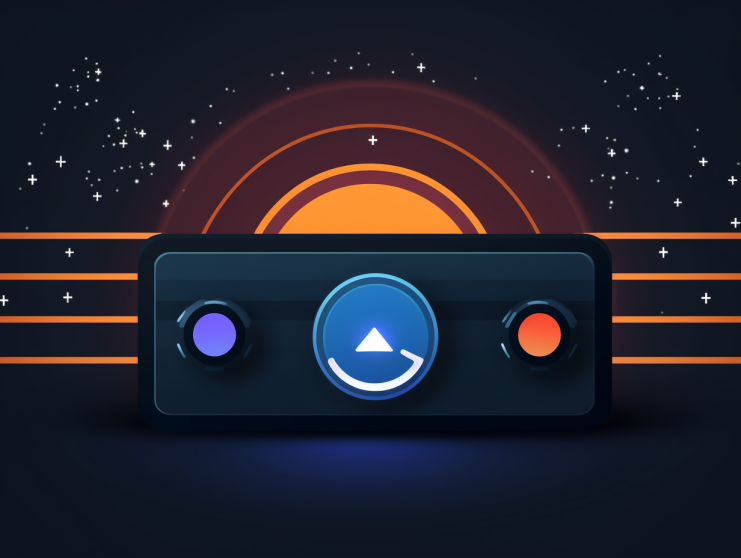
🚦 Laravel Notification Channel Throttling
Throttle your Laravel notifications on a per-channel basis
Introduction
When sending notifications through multiple channels (say email and SMS), you may want to throttle the number of notifications sent through a specific channel. For example, you could limit the number of SMS notifications sent to a user to 1 per day, and limit emails to 5 per day. This package allows you to configure this easily directly in your notification classes.
Installation
You can install the package via composer:
composer require syropian/laravel-notification-channel-throttling
Usage
- Ensure the notification you want to throttle implements
Syropian\LaravelNotificationChannelThrottling\Contracts\ThrottlesChannels
. - Implement the
throttleChannels
method. This method should return an array of channels to throttle, and the configuration for each channel. To omit a channel from throttling either omit the channel from the array, or set the value tofalse
.
use Illuminate\Notifications\Notification; use Syropian\LaravelNotificationChannelThrottling\Contracts\ThrottlesChannels; class ExampleNotification extends Notification implements ThrottlesChannels { // ... public function throttleChannels(object $notifiable, array $channels): array { /** * Throttle the mail channel, so that only one * email notification is sent every 15 minutes */ return [ 'mail' => [ 'maxAttempts' => 1, 'decaySeconds' => 900, ], 'database' => false, ]; } }
Scoping the rate limiter
By default, the rate limiter instance used to throttle is automatically scoped to the notification and channel. If you would like to further scope the rate limiter, you may pass a key
to the channel configuration.
public function __construct(public Post $post) {} public function throttleChannels(object $notifiable, array $channels): array { return [ 'mail' => [ 'key' => $notifiable->id . ':' . $this->post->id, 'maxAttempts' => 1, 'decaySeconds' => 900, ], 'database' => false, ]; }
In this example we're rate limiting the mail channel, and we're scoping it to a specific combination of a user and a post.
Testing
composer test
Credits
License
The MIT License (MIT). Please see License File for more information.