climactic / laravel-credits
A ledger-based Laravel package for managing credit-based systems in your application.
Fund package maintenance!
Climactic
Requires
- php: ^8.4
- illuminate/contracts: ^10.0||^11.0||^12.0
- spatie/laravel-package-tools: ^1.16
Requires (Dev)
- laravel/pint: ^1.14
- nunomaduro/collision: ^8.1.1||^7.10.0
- orchestra/testbench: ^10.0.0||^9.0.0||^8.22.0
- pestphp/pest: ^3.0
- pestphp/pest-plugin-arch: ^3.0
- pestphp/pest-plugin-laravel: ^3.0
This package is auto-updated.
Last update: 2025-08-04 12:52:00 UTC
README
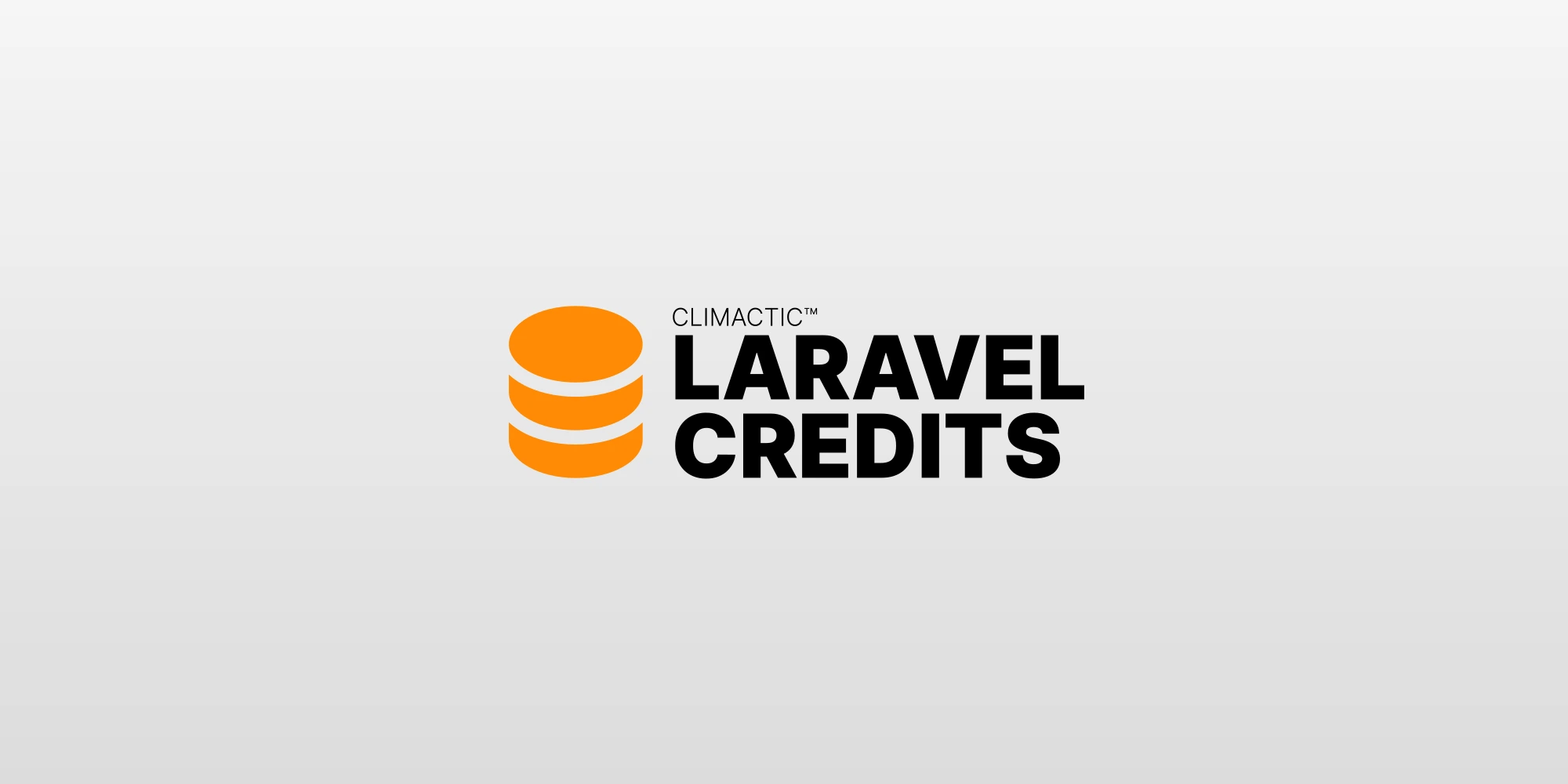
Laravel Credits
A ledger-based Laravel package for managing credit-based systems in your application. Perfect for virtual currencies, reward points, or any credit-based feature.
Features
- 🔄 Credit transactions
- 💸 Credit transfers
- 📢 Events for adding, deducting, and transferring credits
- 💰 Balance tracking with running balance
- 📊 Transaction history
- 🔍 Point-in-time balance lookup
- 📝 Transaction metadata support
- ⚡ Efficient queries using running balance and indexes
Installation
You can install the package via composer:
composer require climactic/laravel-credits
Publish and run the migrations:
php artisan vendor:publish --tag="credits-migrations"
php artisan migrate
Optionally publish the config file:
php artisan vendor:publish --tag="credits-config"
Configuration
return [ // Allow negative balances 'allow_negative_balance' => false, // Table name for credit transactions (change if you've updated the migration table name) 'table_name' => 'credits', ];
Usage
Setup Your Model
Add the HasCredits
trait to any model that should handle credits:
use Climactic\Credits\Traits\HasCredits; class User extends Model { use HasCredits; }
Basic Usage
// Add credits $user->addCredits(100.00, 'Subscription Activated'); // Deduct credits $user->deductCredits(50.00, 'Purchase Made'); // Get current balance $balance = $user->getCurrentBalance(); // Check if user has enough credits if ($user->hasEnoughCredits(30.00)) { // Proceed with transaction }
Transfers
Transfer credits between two models:
$sender->transferCredits($recipient, 100.00, 'Paying to user for their service');
Transaction History
// Get last 10 transactions $history = $user->getTransactionHistory(); // Get last 20 transactions in ascending order $history = $user->getTransactionHistory(20, 'asc');
Historical Balance
Get balance as of a specific date:
$date = new DateTime('2023-01-01'); $balanceAsOf = $user->getBalanceAsOf($date);
Metadata
Add additional information to transactions:
$metadata = [ 'order_id' => 123, 'product' => 'Premium Subscription' ]; $user->addCredits(100.00, 'Purchase', $metadata);
Events
Events are fired for each credit transaction, transfer, and balance update.
The events are:
CreditsAdded
CreditsDeducted
CreditsTransferred
Testing
composer test
Changelog
Please see CHANGELOG for more information on what has changed recently.
Contributing
Please see CONTRIBUTING for details.
Security Vulnerabilities
Please report security vulnerabilities to security@climactic.co.
Sponsors
GitHub Sponsors: @climactic
To become a title sponsor, please contact sponsors@climactic.co.
License
The MIT License (MIT). Please see License File for more information.