capevace / laravel-gpt
A Laravel package for interacting with OpenAI's GPT-3.
Requires
- php: ^8.1
- illuminate/contracts: ^9.0
- orhanerday/open-ai: ^3.2
- spatie/laravel-package-tools: ^1.13.0
Requires (Dev)
- laravel/pint: ^1.0
- nunomaduro/collision: ^6.0
- nunomaduro/larastan: ^2.0.1
- orchestra/testbench: ^7.0
- pestphp/pest: ^1.21
- pestphp/pest-plugin-laravel: ^1.1
- phpstan/extension-installer: ^1.1
- phpstan/phpstan-deprecation-rules: ^1.0
- phpstan/phpstan-phpunit: ^1.0
- phpunit/phpunit: ^9.5
- spatie/laravel-ray: ^1.26
This package is auto-updated.
Last update: 2025-06-09 18:59:04 UTC
README
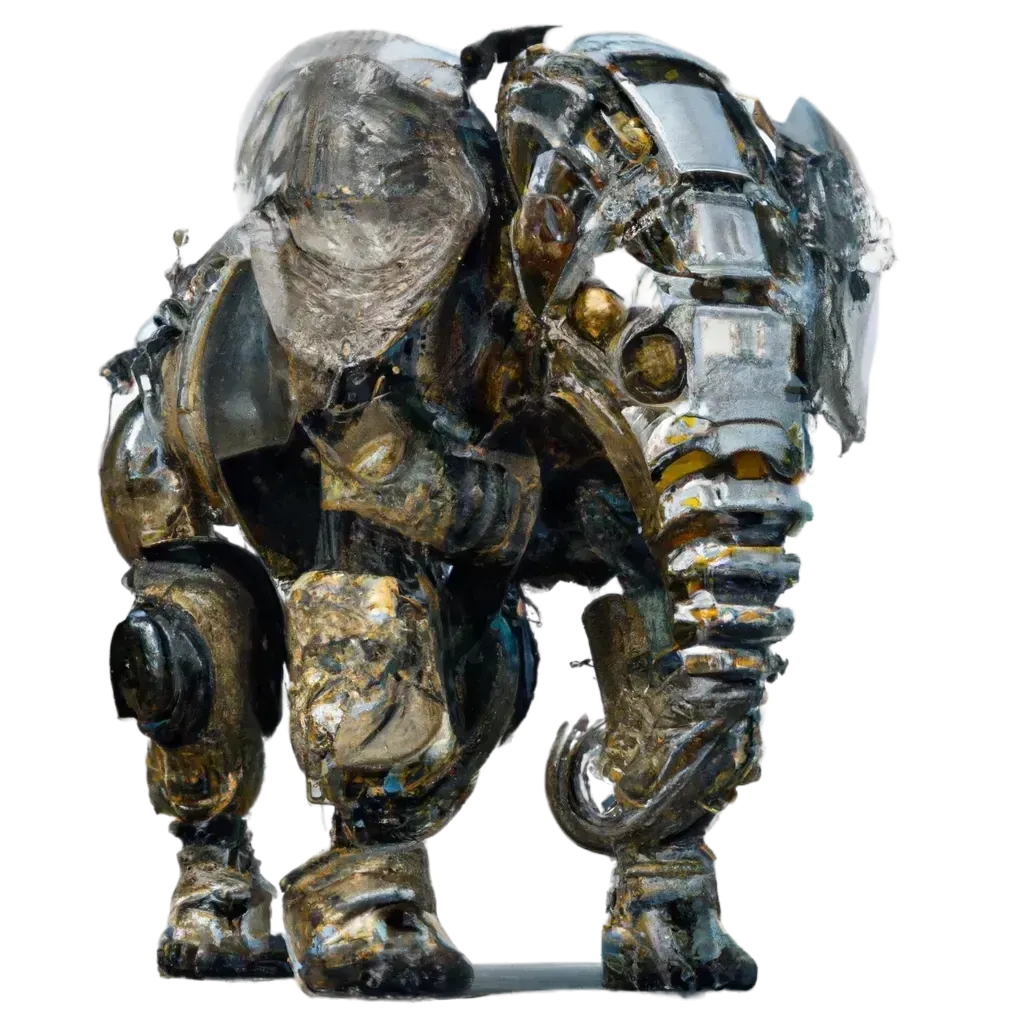
laravel-gpt
This package provides a type-safe interface for making requests to the GPT-3 API.
use Capevace\GPT\Facades\GPT; $response = GPT::generate( 'Name a thing that is blue.', model: 'text-davinci-003', maxTokens: 400, frequencyPenalty: 1.0, ); echo $response->first(); // "The sky"
Installation
You can install the package via composer:
composer require capevace/laravel-gpt
Configuration
You will need an API key for the OpenAI GPT-3 API. Once you have obtained an API key, you can configure it in your .env file by adding the following line:
OPENAI_API_KEY=your-api-key-here
You could also publish the config file directly, but this really probably isn't necessary:
php artisan vendor:publish --tag="laravel-gpt-config"
Usage
The Capevace\GPT\GPTService
class provides methods for making requests to the GPT-3 API. You can inject it into controllers or use the Facade to access the container.
# Access via injection use Capevace\GPT\GPTService; class MyController extends Controller { protected GPTService $gpt; public function __construct(GPTService $gpt) { $this->gpt = $gpt; } public function index() { $this->gpt->generate( // .. ); } } # Access via Facade use Capevace\GPT\Facades\GPT; GPT::generate(/* .. */);
GPT::generate(<prompt>, [...options])
The generate
method returns a GPTResponse
object that contains the response from the GPT-3 API. If no text is returned (empty string), the method will throw an error.
generate
takes the following arguments:
prompt
(required): the prompt to send to the GPT-3 APImodel
: the GPT-3 model to use (defaults to text-davinci-003)temperature
: a value between 0 and 1 that determines how "creative" the response will be (defaults to 0.83)maxTokens
: the maximum number of tokens (i.e., words) to return in the response (defaults to 1200)stop
: a string that, when encountered in the response, will cause the response to end (defaults to null)frequencyPenalty
: a value between 0 and 1 that determines how much the model will penalize frequent words (defaults to 0.11)presencePenalty
: a value between 0 and 1 that determines how much the model will penalize words that don't appear in the prompt (defaults to 0.03)
Example
use Capevace\GPT\Facades\GPT; $response = GPT::generate( 'Generate a list of things that are blue.', model: 'text-davinci-003', maxTokens: 400, frequencyPenalty: 1.0, );
Handling responses
The generate
method returns a GPTResponse
object that contains the response from the GPT-3 API.
It has two methods:
$response->first()
(string): returns the first text suggested by GPT-3$response->all()
(array): returns a list of all the text choices suggested by GPT-3
Example
use Capevace\GPT\Facades\GPT; $response = GPT::generate( 'Name a thing that is blue.', model: 'text-davinci-003', maxTokens: 400, frequencyPenalty: 1.0, ); $firstChoice = $response->first(); // "the sky" $allChoices = $response->all(); // ["the sky", "the ocean" ...]
Error handling
If an error occurs while making a request to the GPT-3 API, the generate()
method will throw a Capevace\GPT\Support\GPTException
exception.
laravel-gpt
will also throw an error, if a response does not contain any text (empty string).
Example
use Capevace\GPT\Facades\GPT; use Capevace\GPT\Support\GPTException; use Exception; try { $response = GPT::generate('Do nothing.'); } catch (GPTException $e) { // Exception will be thrown, as the response text is "" } catch (Exception $e) { // Catch connectivity errors etc. }
Changelog
Please see CHANGELOG for more information on what has changed recently.
License
The MIT License (MIT). Please see License File for more information.