helsingborg-stad / blade
Use Laravel Blade in any PHP project.
Requires
- php: ^7.4|^8.0
- illuminate/view: ^8.0.0
Requires (Dev)
- helsingborg-stad/phpcs: ^0.3.5
- mockery/mockery: ^1.6
- php-mock/php-mock-mockery: ^1.4
- phpunit/phpunit: ^9.6
This package is auto-updated.
Last update: 2025-06-24 15:14:42 UTC
README
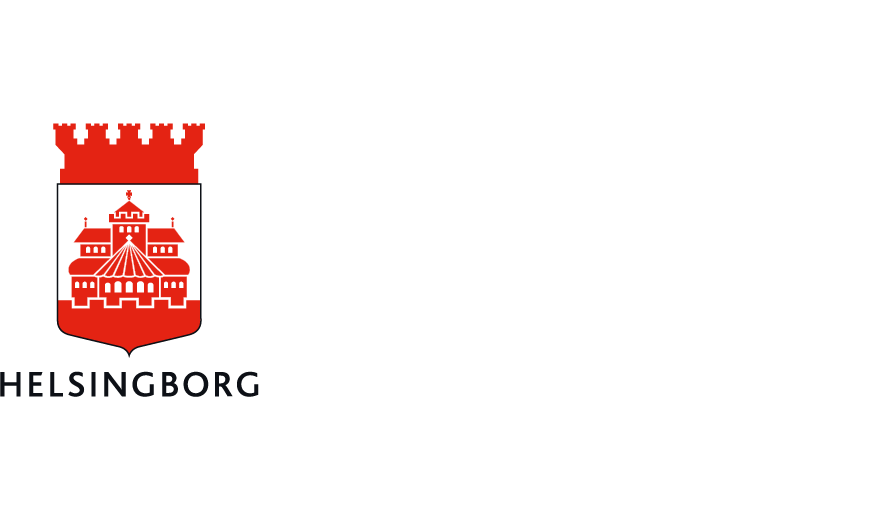
Blade
Use Laravel Blade in any PHP project.
If you don't know about Blade yet, please refer to the official documentation.
Requirements
- PHP ^7.4 | ^8.0
- Composer
Installation
composer require helsingborg-stad/Blade
Usage
Configuration
Cache path
- The cache path can be set by passing the path to the constructor of the BladeService.
- If no cache path is set, the Blade Service will use the system's temporary directory.
- The cache path can be overriden by defining the
BLADE_CACHE_PATH
environment constant.
Initialize blade engine:
This can be done either as a local instance or as a global reusable instance. The global instance is recommended better performance and less memory usage.
Locally
$viewPaths = ['path/to/view/files']; $cachePath = 'path/to/cache/files'; $bladeService = new BladeService($viewPaths, $cachePath);
Globally (for convenient reuse)
$viewPaths = ['path/to/view/files']; $cachePath = 'path/to/cache/files'; $bladeService = GlobalBladeService::getInstance($viewPaths, $cachePath); // You can now reuse the same instance by calling: $sameInstance = GlobalBladeService::getInstance($viewPaths, $cachePath);
Note
If calling GlobalBladeService::getInstance
with parameters after the first call, the $viewPaths will be added and the $cachePath parameter will be ignored.
Render view
$viewFile = 'foo.blade.php'; $html = $bladeService->makeView($viewFile)->render();
Render view with variables
$viewFile = 'foo.blade.php'; $html = $bladeService->makeView($viewFile, ['name' => 'John Doe'])->render();
Render with specific view path
This enables you to temporarily use a specific view path without storing it in the Blade Service.
$viewFile = 'foo.blade.php'; $html = $bladeService->makeView($viewFile, ['name' => 'John Doe'], [], 'specific/view/path')->render();
Register a custom directive
$bladeService->registerDirective('datetime', function ($expression) { return "<?php echo with({$expression})->format('F d, Y'); ?>"; }); // The directive can now be used by adding the @datetime directive to a view file.
Register a component
$bladeService->registerComponent('foo', function ($view) { $view->with('name', 'John Doe'); }); // The component can now be used by adding @component('foo')@endcomponent to a view file.
Register a component directive
If you have already registered a component. That component can be added as a directive by doing the following:
$bladeService->registerComponentDirective('componentname', 'directivename'); // This will register a directive that can be used by adding @directivename()@enddirectivename to a view file, and it will output the component.
Add additional view file paths
If you need to add more view file paths after initializing the Blade Service, this can be done by calling BladeService::addViewPath
$bladeService->addViewPath('extra/view/path');
Prepend view file paths
If you need to add more view file paths before the existing view file paths, this can be done by calling BladeService::prependViewPath
with the second parameter set to true
.
$bladeService->prependViewPath('extra/view/path', true);
Important
For every unique view path added, performance will be affected. This is due to the fact that the Blade Service will have to search through all view paths to find the correct view file. Therefore, it is recommended to add as few view paths as possible.
Error handling
This package offers a convenient solution for swiftly addressing issues that arise within a function called in a view or a syntax error directly within a file. It provides a function to visually display the error, which proves particularly useful during the development process. To optimize development speed and efficiency, it's advisable to implement an error handler when invoking makeView, ensuring smooth troubleshooting whenever errors occur.
try { return $bladeService->makeView($viewFile, ['name' => 'John Doe'], [], 'specific/view/path')->render(); } catch (Throwable $e) { $bladeService->errorHandler($e)->print(); }
Testing
Unit tests
composer test
Code coverage
composer test:coverage