spiral / roadrunner-tcp
RoadRunner: TCP worker
Fund package maintenance!
roadrunner-server
Requires
- php: >=8.1
- ext-json: *
- spiral/roadrunner: ^2023.1 || ^2024.1
- spiral/roadrunner-worker: ^3.0
Requires (Dev)
- jetbrains/phpstorm-attributes: ^1.0
- phpunit/phpunit: ^10.5
- vimeo/psalm: >=5.8
Suggests
- spiral/roadrunner-cli: Provides RoadRunner installation and management CLI tools
README
RoadRunner TCP Plugin
RoadRunner is an open-source (MIT licensed) high-performance PHP application server, load balancer, and process manager. It supports running as a service with the ability to extend its functionality on a per-project basis.
RoadRunner includes TCP server and can be used to replace classic TCP setup with much greater performance and flexibility.
Official Website | Documentation
This repository contains the codebase TCP PHP workers. Check spiral/roadrunner to get application server.
Installation
To install application server and TCP codebase:
composer require spiral/roadrunner-tcp
You can use the convenient installer to download the latest available compatible version of RoadRunner assembly:
composer require spiral/roadrunner-cli --dev
To download latest version of application server:
vendor/bin/rr get
Usage
For example, such a configuration would be quite feasible to run:
tcp: servers: smtp: addr: tcp://127.0.0.1:1025 delimiter: "\r\n" # by default server2: addr: tcp://127.0.0.1:8889 pool: num_workers: 2 max_jobs: 0 allocate_timeout: 60s destroy_timeout: 60s
If you have more than 1 worker in your pool TCP server will send received packets to different workers, and if you need to collect data you have to use storage, that can be accessed by all workers, for example RoadRunner Key Value
Example
To init abstract RoadRunner worker:
<?php require __DIR__ . '/vendor/autoload.php'; use Spiral\RoadRunner\Worker; use Spiral\RoadRunner\Tcp\TcpWorker; use Spiral\RoadRunner\Tcp\TcpResponse; use Spiral\RoadRunner\Tcp\TcpEvent; // Create new RoadRunner worker from global environment $worker = Worker::create(); $tcpWorker = new TcpWorker($worker); while ($request = $tcpWorker->waitRequest()) { try { if ($request->getEvent() === TcpEvent::Connected) { // You can close connection according your restrictions if ($request->getRemoteAddress() !== '127.0.0.1') { $tcpWorker->close(); continue; } // ----------------- // Or continue read data from server // By default, server closes connection if a worker doesn't send CONTINUE response $tcpWorker->read(); // ----------------- // Or send response to the TCP connection, for example, to the SMTP client $tcpWorker->respond("220 mailamie \r\n"); } elseif ($request->getEvent() === TcpEvent::Data) { $body = $request->getBody(); // ... handle request from TCP server [tcp_access_point_1] if ($request->getServer() === 'tcp_access_point_1') { // Send response and close connection $tcpWorker->respond('Access denied', TcpResponse::RespondClose); // ... handle request from TCP server [server2] } elseif ($request->getServer() === 'server2') { // Send response to the TCP connection and wait for the next request $tcpWorker->respond(\json_encode([ 'remote_addr' => $request->getRemoteAddress(), 'server' => $request->getServer(), 'uuid' => $request->getConnectionUuid(), 'body' => $request->getBody(), 'event' => $request->getEvent() ])); } // Handle closed connection event } elseif ($request->getEvent() === TcpEvent::Close) { // Do something ... // You don't need to send response on closed connection } } catch (\Throwable $e) { $tcpWorker->respond("Something went wrong\r\n", TcpResponse::RespondClose); $worker->error((string)$e); } }
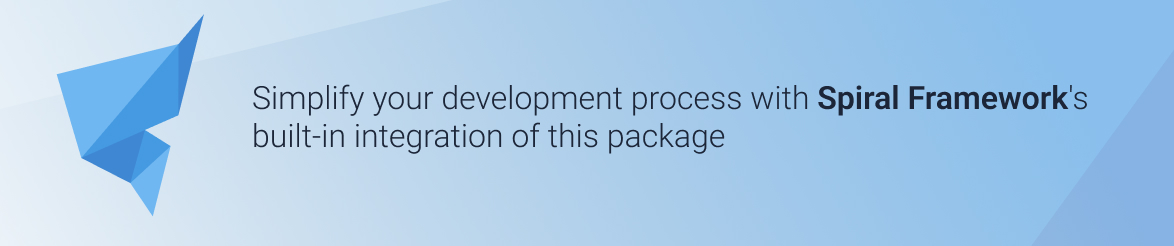
Testing:
This codebase is automatically tested via host repository - spiral/roadrunner.
License:
The MIT License (MIT). Please see LICENSE
for more information. Maintained
by Spiral Scout.