ahmadmayahi / php-amazon-polly
An elegant wrapper around Amazon Polly
Fund package maintenance!
AhmadMayahi
Requires
- php: ^8.1
- aws/aws-sdk-php: ^3.208
Requires (Dev)
- friendsofphp/php-cs-fixer: ^3.0
- phpunit/phpunit: ^9.5
README
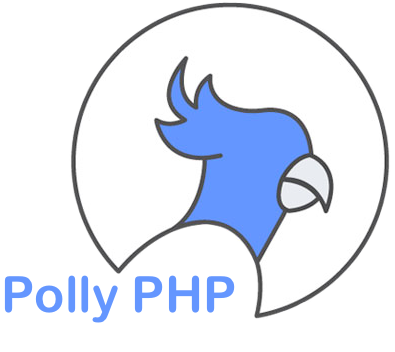
Requires PHP 8.1+
For feedback, please contact me.
PHP Amazon Polly is an easy and elegant wrapper around Amazon Polly, a service that turns text into lifelike speech.
Contents
Installation
You can install the package via composer:
composer require ahmadmayahi/php-amazon-polly
Usage
First you need to configure the client:
use AhmadMayahi\Polly\Config; $config = (new Config()) ->setKey('AWS_KEY') ->setSecret('AWS_SECRET') ->setRegion('eu-west-1'); // default is: us-east-1
Save as MP3 file:
use AhmadMayahi\Polly\Voices\English\UnitedStates; use AhmadMayahi\Polly\Polly; $polly = Polly::init($config); $speechFile = $polly // Desired voice ->voiceId(UnitedStates::Joanna) // Default is MP3. // Available options: asMp3(), asOgg(), asPcm(), asJson() ->asOgg() // Desired Text ->text('Hello World') // Convert and return the object ->convert();
The convert
method returns an object of type AhmadMayahi\Polly\Data\SpeechFile
which has three properties:
file
: the output file asSplFileObject
.speechMarks
(if any).took
: how long did it take to convert the text.
By default, the convert
method saves the file into the default temp. directory; If you want to save the file into a specific directory then you might need to provide the file path as param:
convert('/path/to/desire/file/voice.mp3');
The voiceId()
also accepts a string:
voiceId('Joanna')
Alternatively, you may also specify the output format as an enum
or a string
:
use AhmadMayahi\Polly\Enums\OutputFormat; use AhmadMayahi\Polly\Polly; $polly = Polly::init($config); $speechFile = $polly ->voiceId(UnitedStates::Joanna) // As enum ->outputFormat(OutputFormat::Ogg) // Or as a string ->outputFormat('ogg') ->text('Hello World') ->convert();
Speech Marks
You may also request the Speech Mark Types as follows:
use AhmadMayahi\Polly\Enums\SpeechMarkType; use AhmadMayahi\Polly\Voices\English\UnitedStates; use AhmadMayahi\Polly\Polly; $polly = Polly::init($config); $speechFile = $polly ->voiceId(UnitedStates::Joanna) ->text('Hello World') ->withSpeechMarks(SpeechMarkType::Word, SpeechMarkType::Sentence) ->convert();
Array ( [0] => AhmadMayahi\Polly\Data\SpeechMark Object ( [time] => 0 [type] => AhmadMayahi\Polly\Enums\SpeechMarkType Enum:string ( [name] => Sentence [value] => sentence ) [start] => 7 [end] => 18 [value] => Hello World ) [1] => AhmadMayahi\Polly\Data\SpeechMark Object ( [time] => 6 [type] => AhmadMayahi\Polly\Enums\SpeechMarkType Enum:string ( [name] => Word [value] => word ) [start] => 7 [end] => 12 [value] => Hello ) [2] => AhmadMayahi\Polly\Data\SpeechMark Object ( [time] => 273 [type] => AhmadMayahi\Polly\Enums\SpeechMarkType Enum:string ( [name] => Word [value] => word ) [start] => 13 [end] => 18 [value] => World ) )
SSML
If the given text starts with <spaek>
then the SSML
will be used while synthesizing:
use AhmadMayahi\Polly\Voices\English\UnitedStates; use AhmadMayahi\Polly\Polly; $text = <<<EOL <speak> He was caught up in the game.<break time="1s"/> In the middle of the 10/3/2014 <sub alias="World Wide Web Consortium">W3C</sub> meeting, he shouted, "Nice job!" quite loudly. When his boss stared at him, he repeated <amazon:effect name="whispered">"Nice job,"</amazon:effect> in a whisper. </speak> EOL; $polly = Polly::init($config); $speechFile = $polly ->voiceId(UnitedStates::Ivy) ->text($text) ->convert();
Standard vs Neural Voices
Amazon Polly provides two voice systems Standard
and Neural
.
The Neural
system can produce higher quality voices than the standard voices.
By default, this package will always use the Standard
voice if available, however, some voices such as Olivia
(English Australian) is only available as Neural
.
You may use the neuralVoice()
or standardVoice()
methods as follows:
use AhmadMayahi\Polly\Voices\English\UnitedStates; use AhmadMayahi\Polly\Polly; $polly = Polly::init($config); $speechFile = $polly ->voiceId(UnitedStates::Kendra) ->text('Hello World') ->neuralVoice() ->convert();
Not all the voices support the
nueral
system, for more information please visit Voices in Amazon Polly page.
Convenient Voice Methods
PHP Amazon Polly provides a convenient way to get the appropriate voice id without the need to inspect the documentation.
For example, if you want to use Joanna
you may use englishUnitedStatesJoanna()
method as follows:
use AhmadMayahi\Polly\Polly; $polly = Polly::init($config); $speechFile = $speech ->englishUnitedStatesJoanna($neural = true) ->text('Hello World') ->convert();
As you might have noticed, Joanna
accepts an optional param $neural
, set it to true
if you want neural voice.
Here is the full list of voices with their equivalent method:
Voice | Method |
---|---|
Arabic (Zeina) | arabicZeina() |
Chinese (Zhiyu) | chineseZhiyu() |
Danish (Naja) | danishNaja() |
Danish (Mads) | danishMads() |
Dutch (Lotte) | dutchLotte() |
Dutch (Ruben) | dutchRuben() |
English Australian (Nicole) | englishAustralianNicole() |
English Australian (Olivia) | englishAustralianOlivia($neural = false) |
English Australian (Russel) | englishAustralianRussel() |
English British (Amy) | englishBritishAmy($neural = false) |
English British (Brian) | englishBritishEmma($neural = false) |
English Indian (Aditi) | englishIndianAditi() |
English Indian (Raveena) | englishIndianRaveena() |
English New Zealand (Aria) | englishNewZealandAria() |
English South African (Ayanda) | englishSouthAfricanAyanda() |
English United States (Ivy) | englishUnitedStatesIvy($neural = false) |
English United States (Joanna) | englishUnitedStatesJoanna($neural = false) |
English United States (Kendra) | englishUnitedStatesKendra($neural = false) |
English United States (Kimberly) | englishUnitedStatesKimberly($neural = false) |
English United States (Salli) | englishUnitedStatesSalli($neural = false) |
English United States (Joey) | englishUnitedStatesJoey($neural = false) |
English United States (Justin) | englishUnitedStatesJustin($neural = false) |
English United States (Kevin) | englishUnitedStatesKevin($neural = false) |
English United States (Matthew) | englishUnitedStatesMatthew($neural = false) |
English Welsh (Geraint) | englishWelsh() |
Voice Enums
All the Amazon Polly Voices are supported as enums:
Language | Enum |
---|---|
Arabic | AhmadMayahi\Polly\Voices\Arabic |
Chinese, Mandarin | AhmadMayahi\Polly\Voices\Chinese |
Danish | AhmadMayahi\Polly\Voices\Danish |
Dutch | AhmadMayahi\Polly\Voices\Dutch |
English (Australian) | AhmadMayahi\Polly\Voices\English\Australian |
English (British) | AhmadMayahi\Polly\Voices\English\British |
English (Indian) | AhmadMayahi\Polly\Voices\English\Indian |
English (New Zealand) | AhmadMayahi\Polly\Voices\English\NewZealand |
English (South African) | AhmadMayahi\Polly\Voices\English\SouthAfrican |
English (United States) | AhmadMayahi\Polly\Voices\English\UnitedStates |
French | AhmadMayahi\Polly\Voices\French\French |
French (Canadian) | AhmadMayahi\Polly\Voices\French\Canadian |
German | AhmadMayahi\Polly\Voices\German |
Hindi | AhmadMayahi\Polly\Voices\Hindi |
Icelandic | AhmadMayahi\Polly\Voices\Icelandic |
Italian | AhmadMayahi\Polly\Voices\Italian |
Japanese | AhmadMayahi\Polly\Voices\Japanese |
Korean | AhmadMayahi\Polly\Voices\Korean |
Portuguese (Brazil) | AhmadMayahi\Polly\Voices\Portuguese\Brazil |
Portuguese (Portugal) | AhmadMayahi\Polly\Voices\Portuguese\Portugal |
Romanian | AhmadMayahi\Polly\Voices\Romanian |
Russian | AhmadMayahi\Polly\Voices\Russian |
Spanish (Mexican) | AhmadMayahi\Polly\Voices\Spanish\Mexico |
Spanish (Spain) | AhmadMayahi\Polly\Voices\Spanish\Spain |
Spanish (United States) | AhmadMayahi\Polly\Voices\Spanish\UnitedStates |
Swedish | AhmadMayahi\Polly\Voices\Swedish |
Turkish | AhmadMayahi\Polly\Voices\Turkish |
Welsh | AhmadMayahi\Polly\Voices\Welsh |
For example, if you want to get Nicole
from English (Australian):
use AhmadMayahi\Polly\Voices\English\Australian; Australian::Nicole;
Describe Voice
You can also describe the voice using the describe
method as follows:
use AhmadMayahi\Polly\Voices\English\Australian; Australian::Nicole->describe();
The describe
method returns an object of type AhmadMayahi\Polly\Data\DescribeVoice
with the following properties:
gender
: Determines the voice's gender.neural
: Is it Neural Voice?standard
: Is it Standard Voice?bilingual
: Is it bilingual? Read more.newscaster
: Does it do a newscaster speaking style? Read morechild
: Child speaker?
According to Amazon Polly documentation, Aditi (Hindi) she's the only one who speaks both Indian English (en-IN) and Hindi (hi-IN) fluently.
Testing
composer test
Changelog
Please see CHANGELOG for more information on what has changed recently.
Contributing
Please see CONTRIBUTING for details.
Security Vulnerabilities
Please review our security policy on how to report security vulnerabilities.
Credits
License
The MIT License (MIT). Please see License File for more information.