kssadi / sslwirelesssms
A Laravel package to integrate SSL Wireless SMS service.
Requires
- php: ^8.0
README
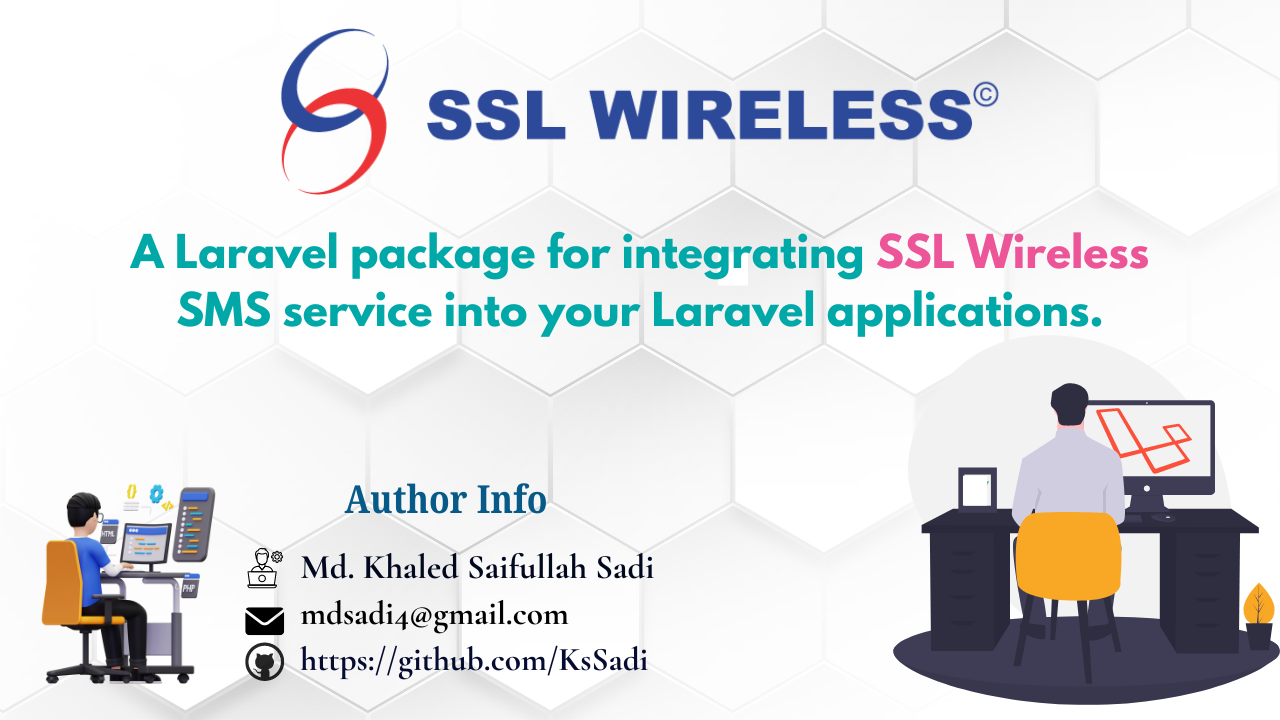
SSL Wireless SMS Laravel Package
A Laravel package for integrating SSL Wireless SMS service into your Laravel applications. Easily send single, bulk, and dynamic SMS messages using this package.
Features
- Single SMS: Send a single SMS message to a phone number.
- Bulk SMS: Send SMS messages to multiple phone numbers in a single request.
- Dynamic SMS: Send SMS messages dynamically with varying content.
Table of Contents
Requirements
- PHP >= 8.0
- Laravel >= 10
Installation
-
Install the package via Composer:
composer require kssadi/sslwirelesssms
-
Publish the configuration file:
php artisan vendor:publish --provider="KsSadi\SSLWirelessSMS\SSLWirelessSMSServiceProvider" --tag="config"
This will publish the
sslwireless.php
configuration file to yourconfig
directory.
Configuration
Add your SSL Wireless SMS credentials to the config/sslwireless.php
file
return [ 'api_token' => env('SSLWIRELESS_API_TOKEN', ''), 'sid' => env('SSLWIRELESS_SID', ''), 'domain' => env('SSLWIRELESS_DOMAIN', 'https://smsplus.sslwireless.com'), 'message_type' => env('SSLWIRELESS_MESSAGE_TYPE', 'EN'), ];
Add these values to your .env
file:
SSLWIRELESS_API_TOKEN=your_api_token SSLWIRELESS_SID=your_sid
Usage
With this package, you can send single, bulk, or dynamic SMS messages using a unified method sendSms(). This method simplifies the process by handling all types of SMS through a single function. If you prefer, you can also use separate methods for each type of SMS.
Unified SMS Sending
The sendSms()
method allows you to send different types of SMS with a single function, depending on the data you provide:
Single SMS: Send a single SMS message to one phone number.
Bulk SMS: Send the same SMS message to multiple phone numbers.
Dynamic SMS: Send different SMS messages to multiple phone numbers.
Example: Sending Single SMS
use KsSadi\SSLWirelessSMS\Facades\SSLWirelessSMS; $response = SSLWirelessSMS::sendSms([ 'phoneNumber' => '1234567890', 'messageBody' => 'Hello World' ], 'txn123');
Example: Sending Bulk SMS
use KsSadi\SSLWirelessSMS\Facades\SSLWirelessSMS; $response = SSLWirelessSMS::sendSms([ 'phoneNumbers' => ['1234567890', '0987654321'], 'messageBody' => 'Hello, this is a test message for bulk SMS.' ], 'batch123');
Example: Sending Dynamic SMS
use KsSadi\SSLWirelessSMS\Facades\SSLWirelessSMS; $response = SSLWirelessSMS::sendSms([ 'messages' => [ ['phoneNumber' => '1234567890', 'message' => 'Hello, User 1!', 'sms_id' => 'sms1'], ['phoneNumber' => '0987654321', 'message' => 'Hello, User 2!', 'sms_id' => 'sms2'] ] ]);
Separate Methods
If you prefer to use separate methods for each type of SMS, you can do so as follows:
sendSingleSms()
: Send a single SMS message.
sendBulkSms()
: Send SMS messages to multiple phone numbers.
sendDynamicSms()
: Send different SMS messages to multiple phone numbers.
Example: Sending Single SMS
use KsSadi\SSLWirelessSMS\Facades\SSLWirelessSMS; $response = SSLWirelessSMS::sendSingleSms('1234567890', 'Hello, world!', 'TX123');
Example: Sending a Bulk SMS
use KsSadi\SSLWirelessSMS\Facades\SSLWirelessSMS; $phoneNumbers = ['1234567890', '0987654321']; $response = SSLWirelessSMS::sendBulkSms($phoneNumbers, 'Hello, world!', 'TX123');
Example: Sending a Dynamic SMS
use KsSadi\SSLWirelessSMS\Facades\SSLWirelessSMS; $messageData = [ [ 'phone_number' => '1234567890', 'message' => 'Hello, John!', "sms_id" => uniqid() //must be unique ], [ 'phone_number' => '0987654321', 'message' => 'Hello, Jane!', "sms_id" => uniqid() //must be unique ] ]; $response = SSLWirelessSMS::sendDynamicSms($messageData);
Author
Name: Khaled Saifullah Sadi
Email: mdsadi4@gmail.com
Social Handles
Contributing
Please feel free to contribute to this package and submit a pull request.
License
This package is open-source software licensed under the MIT license.
Key Sections:
- Features: Highlights the capabilities of the package.
- Table of Contents: Provides an overview for easy navigation.
- Installation: Instructions to install the package.
- Configuration: Details on setting up configuration.
- Usage: Examples of how to use the package functions.
- Testing: Instructions for running tests.
- Author: Information about the package author.
- Contributing: Guidelines for contributing to the project.
- License: Information about the package's licensing.
Feel free to adjust the content as needed based on your specific use cases or additional features.
Copyright 2024 Khaled Saifullah Sadi