gostellarco / nylas-php
Nylas PHP SDK (api version 2.1)
Requires
- php: >=7.4
- ext-json: *
- guzzlehttp/guzzle: >=6.5
- respect/validation: ^2.0
- zbateson/mail-mime-parser: ^1.2
Requires (Dev)
- phpunit/phpunit: ^9.2
README
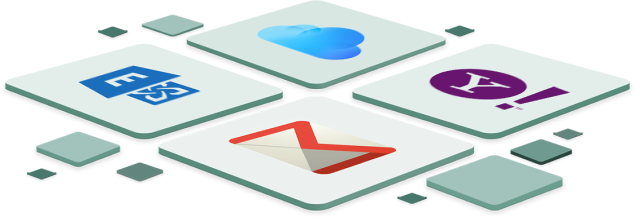
Nylas PHP SDK
PHP bindings for the Nylas REST API (V2.1). https://docs.nylas.com/reference
I'll try to keep up with NyLas Changelog in future updates.
Last check at the point: Dashboard Release for Canada and Ireland
What's new?
- API 2.1 support
- All Nylas APIs have been implemented within this SDK.
- Support send & get message in raw type
- Support async batch upload & download
-- Contact picture download
-- File upload & download - The parameters that required by methods almost the same as nylas official api required.
- Support async batch get & delete & send (since version 3.1).
- Chained calls and good code hints, easy to use
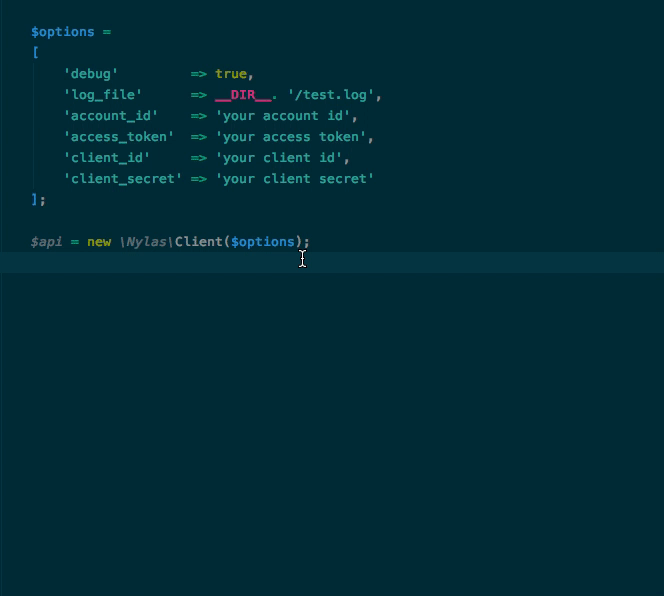
Installation (PHP 7.4 required since version 4.0)
version 3.x for php >= 7.3 (branch 3.0)
version 4.x for php >= 7.4 (branch master)
This library is available on https://packagist.org/packages/lanlin/nylas-php
You can install it by running
composer require lanlin/nylas-php
Usage
App ID and Secret
Before you can interact with the Nylas REST API,
you need to create a Nylas developer account at https://www.nylas.com/.
After you've created a developer account, you can create a new application to generate an App ID / Secret pair.
Generally, you should store your App ID and Secret into environment variables to avoid adding them to source control.
The test projects use configuration files instead, to make it easier to get started.
Init Nylas-PHP
TIPS: 'off_decode_error' has removed since version 4.1.2, see Error & Exceptions
use Nylas\Client; $options = [ 'debug' => true, 'region' => 'oregon', // server region, can be oregon, ireland or canada, default is oregon 'log_file' => dirname(__FILE__) . '/test.log', // a file path or a resource handler 'account_id' => 'your account id', 'access_token' => 'your access token', 'client_id' => 'your client id', // required 'client_secret' => 'your client secret' // required ]; $nylas = new Client($options);
Options Setting
You can modify options with these methods:
$nylas->Options()->
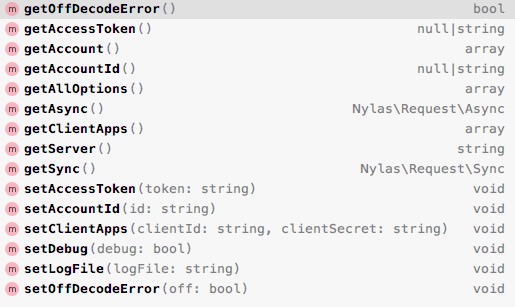
Batch Request
Most of the methods that have the get & delete prefix support batch request.
$id = 'id_xxx'; $ids = ['id_xxx', 'id_yyy', ...]; // one per time $dataA = $nylas->Contacts()->Contact()->getContact($id); $dataB = $nylas->Contacts()->Contact()->deleteContact($id); // batch request $dataC = $nylas->Contacts()->Contact()->getContact($ids); $dataD = $nylas->Contacts()->Contact()->deleteContact($ids);
For more detail about the batch request, you should have to read the source code.
Sorry, I have no time to write documents.
Authentication
There are two ways you can authenticate users to your application.
Hosted & Native are both supported.
Here's the server-side(three-legged) OAuth example:
- You redirect the user to nylas login page, along with your App Id and Secret
- Your user logs in
- She is redirected to a callback URL of your own, along with an access code
- You use this access code to get an authorization token to the API
For more information about authenticating with Nylas,
visit the Developer Documentation.
In practice, the Nylas REST API client simplifies this down to two steps.
Step 1: Redirect the user to Nylas:
$params = [ 'state' => 'testing', 'login_hint' => 'test@gmail.com', 'redirect_uri' => 'https://www.test.com/redirect_callback', ]; // generate the url that your user need be redirect to. $url = $nylas->Authentication()->Hosted()->getOAuthAuthorizeUrl($params);
Step 2: your user logs in:
Step 3: you got the access code from the nylas callback:
Please implement the above 2 & 3 steps yourself.
Step 4: Get authorization token with access code:
$data = $nylas->Authentication()->Hosted()->postOAuthToken($params); // save your token some where // or update the client option $nylas->Options()->setAccessToken("pass the token you got");
Error & Exceptions
-
common error codes that response from nylas are wrapped as exceptions, (see
src/Exceptions
) and the exception code is the same as nylas api error list -
you will get an array like below, when response data was not a valid json string or even not json content type:
[ 'httpStatus' => 'http status code', 'invalidJson' => true, 'contentType' => 'response header content type', 'contentBody' => 'response body content', ]
-
for all methods that execute as the async mode will not throw an exception when an error occurs, instead, it will return an array which contains all data and exceptions inside like below:
[ // ... [ 'error' => true, 'code' => 'exception code', 'message' => 'exception message', 'exception' => 'exception instance', ], // ... ]
-
some email provider may not support all features, exp: calendar, event. for that reason you may get an exception named
BadRequestException
with 400 code and the msg:Malformed or missing a required parameter, or your email provider not support this.
-
the
log_file
parameter only works whendebug
set totrue
, then the detailed info of the http request will be logged.Tips: nylas-php use the guzzlehttp for http request. but guzzlehttp only support a resource type as the debug handler (cURL CURLOPT_STDERR required that).
for anyone who wants to use psr/log interface to debug, you can init a temp resource, and pass the handler to nylas-php, then get log content from temp resource after calling some methods.
$handler = fopen('php://temp', 'w+'); $options = [ 'log_file' => $handler, ... ]; $nylas = new Client($options); $nylas->doSomething(); .... rewind($handler); $logContent = stream_get_contents($handler); fclose($handler); $yourPsrLogger->debug($logContent);
Launching the tests
- Initialise composer dependency
composer install
- Add your info in
tests/AbsCase.php
- Launch the test with
composer run-script test
- Another way to run tests:
./tests/do.sh foo.php --filter fooMethod
, seetests/do.sh
Supported Methods
The parameters that required by methods almost the same as nylas official api required.
For more detail, you can view the tests or the source code of validation rules for that method.
Accounts
$nylas->Accounts()->Account()->xxx(); $nylas->Accounts()->Manage()->xxx();
Authentication
$nylas->Authentication()->Hosted()->xxx(); $nylas->Authentication()->Native()->xxx();
Calendars
$nylas->Calendars()->Calendar()->xxx();
Contacts
$nylas->Contacts()->Contact()->xxx();
// multiple contact pictures download $params = [ [ 'id' => 'contact id', 'path' => 'this can be a file path, resource or stream handle', ], [ 'id' => 'xxxx', 'path' => dirname(__FILE__) . '/correct.png', ], // ... ]; $nylas->Contacts()->Contact()->getContactPicture($params);
Deltas
$nylas->Deltas()->Delta()->xxx();
Draft
$nylas->Drafts()->Draft()->xxx(); $nylas->Drafts()->Sending()->xxx();
Events
$nylas->Events()->Event()->xxx();
Files
$nylas->Files()->File()->xxx();
// multiple files download $params = [ [ 'id' => 'file id', 'path' => 'this can be a file path, resource or stream handle', ], [ 'id' => 'xxxx', 'path' => dirname(__FILE__) . '/correct.png', ], // ... ]; $nylas->Files()->File()->downloadFile($params); // multiple files upload $params = [ [ 'contents' => 'this can be a file path, resource or stream handle', 'filename' => 'your file name' ], [ 'contents' => dirname(__FILE__) . '/correct.png', 'filename' => 'test_correct.png' ], // ... ]; $nylas->Files()->File()->uploadFile($params);
Folders
$nylas->Folders()->Folder()->xxx();
Labels
$nylas->Labels()->Label()->xxx();
Job-Statuses
$nylas->JobStatuses()->JobStatus()->xxx();
Messages
$nylas->Messages()->Message()->xxx(); $nylas->Messages()->Search()->xxx(); $nylas->Messages()->Sending()->xxx(); $nylas->Messages()->Smart()->xxx();
Threads
$nylas->Threads()->Search()->xxx(); $nylas->Threads()->Thread()->xxx(); $nylas->Threads()->Smart()->xxx();
Webhooks
$nylas->Webhooks()->Webhook()->xxx();
Contributing
For more usage demos, please view the tests.
Please feel free to use it and send me a pull request if you fix anything or add a feature, though.
License
This project is licensed under the MIT license.