fruitsbytes / php-moncash
PHP library to use Digicel MonCash
Requires
- php: >=8.0
- ext-bcmath: *
- ext-curl: *
- ext-json: *
- ext-openssl: *
- giggsey/libphonenumber-for-php: ^8.12
- ramsey/uuid: ^4.5
- vlucas/phpdotenv: ^5.5
Requires (Dev)
- jetbrains/phpstorm-attributes: ^1.0
- phpunit/phpunit: ^9.5.26
This package is auto-updated.
Last update: 2025-06-12 03:51:04 UTC
README
A library to facilitate Digicel MonCash mobile money integration on your PHP projects via their API. For now it handles the base (client) use cae. The merchant use cases wil be available soon. It is part of the MonCash SDK provided by FruitsBytes.
🍇Features
- Client side Adapter (Payment, Transfer, ...)
- Render Button
- Payment
- Transfer
- Traffic optimisation (
Advanced
) - Security: Secret Management (
Advanced
) - Unique orderID generator
- Retry (
Advanced
) - Phone Validation (
Advanced
) - Client Button, No Backend (Encrypted, client side button)
- Merchant App (Cash-In , Cash-Out, ...)
- Idempotence (
Advanced
) - Localization (
Advanced
) - Task (Recurring payments, garbage collector,...)
Check the CHANGELOG for additional information on breaking changes and new features.
🍈Documentation
For a complete guide please check the Wiki or the code examples.
🍉Quick Start
Installation
composer require fruitsbytes/php-moncash
Configuration
// index.php use Fruitsbytes\PHP\MonCash\API\Client; $client = new Client( [ 'clientSecret' => 'my-client-secret', 'clientId' => 'my-client-key', 'businessKey' => 'my-merchant-key', ] );
You can update or set the configuration:
use Fruitsbytes\PHP\MonCash\Configuration\Configuration; $configuration = new Configuration(["lang"=>"ht"]); $client = new Client($configuration);
The default no config value :
// src/Configuration/Configuration.php const DEFAULT_CONFIG = [ 'mode' => 'sandbox', 'lang' => 'en', 'clientSecret' => '', 'clientId' => '', 'businessKey' => '', 'rsaPath' => './rsa.text', 'timeout' => 60, 'secretManager' => DefaultSecretManager::class, 'tokenMachine' => FileTokenMachine::class, 'phoneValidation' => LibPhoneValidation::class, 'orderIdGenerator' => UUIDOrderIdGenerator::class, ];
Usage
Client
For client facing websites and mobile app, where the client iniates the payment.
// Create a payment & redirect user use Fruitsbytes\PHP\MonCash\API\Order; use Fruitsbytes\PHP\MonCash\API\PaymentFoundResponse; use Fruitsbytes\PHP\MonCash\API\PaymentFoundResponse; use Fruitsbytes\PHP\MonCash\APIException; use Exception; try{ $order = new Order($amount); // if you have your own orderID `new Order($amount, $uuid)` $payment = $client->createAndRedirect($order); }catch( APIException $e){ $message = $e->getMessage(); } catch( Exception $e){ $message = $e->getMessage(); } // get payment by orderID if ypui do not habe the transation ID yet /** * @var PaymentFoundResponse */ $paymentResult = $client->getPaymentByOrderId($order->id); // get payment by transactionID when transaction is finished $transactionId = $_GET['transactionId'] /** * @var PaymentFoundResponse */ $paymentResult = $client->getPaymentByTransactionId($transactionId); if(!$payment->isSuccessful()){ throw new \Exception($payment->message); }
Button
The button, when clicked, will create the transaction and redirects the user to the
// Generate Button form html code use Fruitsbytes\PHP\MonCash\Button\ButtonStyleRedResponsive; $buttonConfig = [ true, // border 'em', // lang true, // animate on hover, 48 // height ]; $button = new ButtonStyleRedResponsive( $order, $clientConfig, ...$buttonConfig); $htmlButton = $button->html(); print($htmlButton); // or Use the \Stringable interface print $button;
You can render the template directly
$buttonHT = new ButtonStyleRedResponsive( $order, [], true, 'ht'); $buttonHT->render();
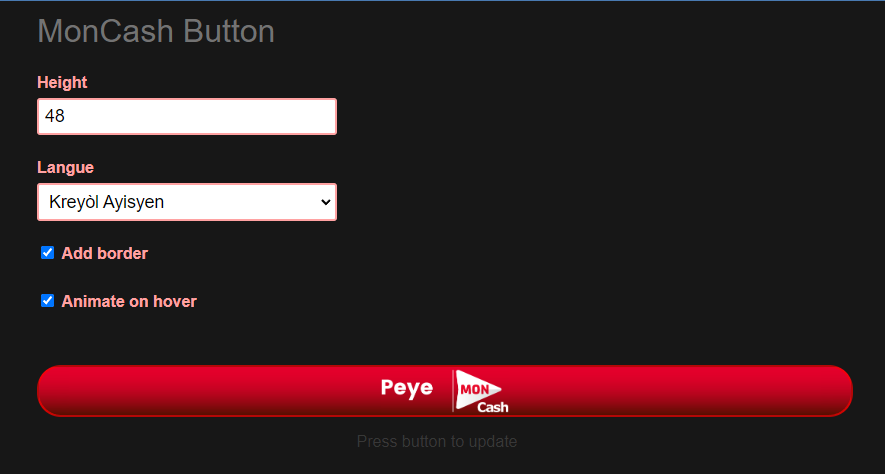
Button config Playground
Merchant
For applications aimed at store clerks or business owners where the client is present at the time of the transaction. It handles Cash-In and Cash-Out scenarios.
==== 🚧 Coming soon =====
🍊Playground
==== 🚧 Website Coming soon =====
You can check the Postman API online or import the .json from this repository.
🍍Contribution
All pull requests and suggestions are welcomed. If there is a particular feature you are struggling make a pull reque
🍒Test
When modifyiing To run the test use the following shell command from this directory.
Set the values of .env.testing
environment file.
composer phpunit
or
vendor\bin\phpunit
⚠ Reduce credentials being leaked by not using production credentials for testing.
🍓Other
FruitsBytes MonCash SDK:
Repo | Description | Version | Status |
---|---|---|---|
Laravel examples | Examples using pure laravel codes, no- external libraries. | ✅ | |
Laravel | A fully funtional package for laravel | alpha not ready to share |
🚧 |
Wordpress | Use MonCash on Wordpress sites. Also available for Woocommerce integration | alpha not ready to share |
🚧 |
Shopify | Use MonCash as a shopify payment method | alpha not ready to share |
🚧 |
NodeJS | Using NodeJS as a server this can be used with idependently or as an API (for non-SSR websites for example). | alpha not ready to share |
🚧 |
Javascript Examples | Repositories with a general idea on how to consume the API with several use case and JS Frameworks native solution | alpha not ready to share |
🚧 |
Angular | Configurable Button + Server side compolnents (Angular Universal) | alpha not ready to share |
🚧 |
ReactJS | Configurable Button + Server side compolnents (Next.js, ) | alpha not ready to share |
🚧 |
VueJS | Configurable Button | alpha not ready to share |
🚧 |
Capacitor | IonicFramework Plugin for Android and IOS with deepLink integration support and extra security | alpha not ready to share |
🚧 |
MonCash Documentation
- Client Rest API ( client facing user interface)
- Merchant Rest API (admin/merchant facing UI)
- Dashboard: sandbox (for test) | production (live)
Offical Repo
- ecelestin/ ecelestin-Moncash-sdk-php ✍ ing. Enadyre celeste
Online videos :
Title | Link |
---|---|
Kijan pou mete Moncash sou sit ou pou w vann (🇭🇹) ✍ Certil Rémy |
|
Comment Intégrer l'onglet Moncash Pay à votre commerce online - Technopro Web (🇫🇷) ✍ Osirus Kurt, RIP 🕊 |
🍋Security
If you discover a security vulnerability within this package, please send an email to security@anbapyezanman.com. All security vulnerabilities will be addressed as soon as possible. You may view our full security policy here.
🍎Need help?
Don't hesitate to go in the discussion page and check if the issue is not addressed yet. You can start a new discussion if need be.
If you are an upcoming startup, a student or don't have the budget for consultation fees, it will take longer but we can submit a public repo illustrating the help you need as long as it will benefit the rest of the community:
Contact us at help@anbapyezanman.com
![]() |
You can also check our Youtube Channel |
For Enterprise
Fruitsbytes can deliver commercial support and maintenance for your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use.
Contact us at business@anbapyezanman.com
🍏License
This library is licensed under The MIT License.
Discalimer
* The Digicel™, MonCash™, Sogebank™ and all other trademarks, logos and brand names are the property of their respective owners. All company, product and service names used in this documentation are for identification purposes only. Use of these names,trademarks and brands does not imply endorsement.
MonCash™ is a mobile money service provided by Digicel™ that allows daily transactions between MonCash users, regardless of their location in Haiti. Digicel is a pioneer in mobile money. Their financial services are currently expanding into other markets, specifically in the pacific island with MyCash™ [1] [2]