linna / typed-array
Linna Typed Array, typed arrays for PHP
Installs: 6 636
Dependents: 2
Suggesters: 0
Security: 0
Stars: 7
Watchers: 3
Forks: 3
Open Issues: 1
Requires
- php: >=8.1
Requires (Dev)
- infection/infection: >=0.26
- phpstan/phpstan: ^1.9
- phpunit/phpunit: ^10
This package is auto-updated.
Last update: 2025-07-11 01:06:42 UTC
README
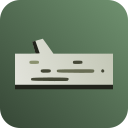

About
This package provide typed arrays for php as extension of native ArrayObject.
Requirements
This package require php 8.1
Installation
With composer:
composer require linna/typed-array
Classes
Name | Native Type Handled | Description |
---|---|---|
ArrayOfArrays |
array | |
ArrayOfBooleans |
bool | |
ArrayOfCallable |
callable | |
ArrayOfClasses |
any existing class | passed as first argument in constructor as ::class |
ArrayOfFloats |
float | |
ArrayOfIntegers |
int | |
ArrayOfObjects |
object | |
ArrayOfStrings |
string |
Usage
use Linna\TypedArrayObject\ArrayOfIntegers; use Linna\TypedArrayObject\ArrayOfClasses; //correct, only int passed to constructor. $intArray = new ArrayOfIntegers([1, 2, 3, 4]); //correct, int assigned $intArray[] = 5; //throw InvalidArgumentException, string assigned, int expected. $intArray[] = 'a'; //correct, int used $intArray->append(5); //throw InvalidArgumentException, string used, int expected. $intArray->append('a'); //throw InvalidArgumentException, mixed array passed to constructor. $otherIntArray = new ArrayOfIntegers([1, 'a', 3, 4]); //correct, only Foo class instances passed to constructor. $fooArray = new ArrayOfClasses(Foo::class, [ new Foo(), new Foo() ]); //correct, Foo() instance assigned. $fooArray[] = new Foo(); //throw InvalidArgumentException, Bar() instance assigned. $fooArray[] = new Bar(); //correct, Foo() instance used. $fooArray->append(new Foo()); //throw InvalidArgumentException, Bar() instance used, Foo() instance expected. $fooArray->append(new Bar()); //throw InvalidArgumentException, mixed array of instances passed to constructor. $otherFooArray = new ArrayOfClasses(Foo::class, [ new Foo(), new Bar() ]);
Note: Allowed types are: array, bool, callable, float, int, object, string and all existing classes.
Performance consideration for v3.0
Compared to previous versions of the library, this version is a bit faster because every types has it own class. Do milliseconds really matters?
Performance consideration for v2.0
Compared to first version of the library, this version is a bit slower because after merging TypedObjectArray
with TypedArray
,
there are more code that be executed when new instance is created and on assign operations.
Performance consideration for v1.0
Compared to the parent class ArrayObject typed arrays are slower on writing
approximately from 6x to 8x. The slowness is due to not native __construct()
and not native offsetSet()
.
Other operations do not have a speed difference with the native ArrayObject.
use Linna\TypedArray; //slower from 6x to 8x. $array = new TypedArray('int', [1, 2, 3, 4]); $array[] = 5; //other operations, fast as native. //for example: $arrayElement = $array[0]; $elements = $array->count();
View the speed test script on gist.