linna / csrf-guard
Linna Cross-site request forgery Guard
Requires
- php: >=8.1
Requires (Dev)
- infection/infection: >=0.26
- phpstan/phpstan: ^1.9
- phpunit/phpunit: ^10
This package is auto-updated.
Last update: 2025-06-11 14:13:28 UTC
README
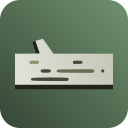

About
Provide a class for generate and validate tokens utilized against Cross-site Request Forgery.
Note: Don't consider this class a definitive method to protect your web site/application. If you wish deepen how to prevent csrf you can start here
Requirements
This package require
- php 7.0 until version v1.1.2
- php 7.1 from v1.2.0
- php 7.4 from v1.4.0
- php 8.1 from v2.0.0
Installation
With composer:
composer require linna/csrf-guard
Token types
Note: Storage it's intended that the data about token or the token is stored in session.
The package provides three types of token:
- Encryption-based CSRF token
- HMAC-based CSRF token
- Synchronizer CSRF token
Encryption-based token
Encryption-based CSRF token is a token that is the result of a cryptographic algorithm, some data is encrypted using a
secret key only known from the server .The implementation in this library uses libsodium
aead contruction
XChaCha20-Poly1305
. The token has expire time and require local storage.
The token security depends from:
- secret key storage
- strength of
XChaCha20-Poly1305
This token is valid until validated or until it expires. It's possible to select a length of the token. The length of the token doesn't affect the storage used.
The key used for the engryption is generated for every session, the nonce for every token.
HMAC-based token
HMAC-based CSRF token is a token that is computed by applying an HMAC function to some data and a secret key that is
only known from the server. The implementation in this library uses php hash_hmac
with the sha3-384
algorithm.
This type of token deosn't require local storage and it has an expire time.
The token security depends from:
- secret key storage
- strength of
sha3-384
This token is valid until expires and can be validate more times. Also has fixed length and it's not possible to change it to obtain a shorter or longer token.
The key used to authenticate is fully managed by the user of the library.
Synchronizer token
The Synchronizer CSRF token is a token randomly generated. This library uses php random_bytes
. The token has expire
time and require local storage.
The token security depends from:
- the length of the token
This token is valid until validated or until it expires. It's possible to select a length of the token. The length of the token affects the storage used.
Usage
Note: Session must be started before you create the instance of a provider, if no a
SessionNotStartedException
will be throw, this is not true if you use theHmacTokenProvider
.
Get started
How to get and validate a token using few lines of code.
Generate a provider
//start the session \session_start(); //generate token provider $provider = ProviderSimpleFactory::getProvider();
Get a token
//previous php code //get a token from provider $token = $provider->getToken();
Validate it
//previous php code //true if valid, false otherwise $isValid = $provider->validate($token);
Provider configuration
The ProviderSimpleFactory::getProvider()
static method has two parameters:
- the provider
- options for the provider
EncryptionTokenProvider config
Options | Default Value | Unity | Range | Mandatory |
---|---|---|---|---|
expire | 600 | seconds | 0-86400 | no |
storageSize | 10 | tokens | 2-64 | no |
tokenLength | 16 | bytes | 16-128 | no |
Example of usage:
//start the session \session_start(); //get specific encryption token provider $provider = ProviderSimpleFactory::getProvider( provider: EncryptionTokenProvider::class, // specific token provider options: [ // options 'expire' => 3600, // token expire in 3600 seconds, 1 hour 'storageSize' => 16, // provider can store maximum 1 key and 16 nonces per session, 'tokenLength' => 16 // desidered token length in bytes, token will be used as plaintext and not stored ] );
HmacTokenProvider config
Options | Default Value | Unity | Range | Mandatory |
---|---|---|---|---|
value | // | yes | ||
key | // | yes | ||
expire | 600 | seconds | 0-86400 | no |
Example of usage:
//get specific hmac token provider $provider = ProviderSimpleFactory::getProvider( provider: HmacTokenProvider::class, // specific token provider options: [ // options 'value' => 'value will be hashed in token', // value will be hashed in token 'key' => 'key_to_authenticate' // key to authenticate the hash ] );
SynchronizerTokenProvider config
Options | Default Value | Unity | Range | Mandatory |
---|---|---|---|---|
expire | 600 | seconds | 0-86400 | no |
storageSize | 10 | tokens | 2-64 | no |
tokenLength | 32 | bytes | 16-128 | no |
Example of usage:
//start the session \session_start(); //get specific syncronizer token provider $provider = ProviderSimpleFactory::getProvider( provider: SynchronizerTokenProvider::class, // specific token provider options: [ // options 'expire' => 3600, // token expire in 3600 seconds, 1 hour 'storageSize' => 16, // provider can store maximum 16 token per session, 'tokenLength' => 32 // desidered token length in bytes, token will be the double in chars ] );