kmuharam / tamex-php
This project is a PHP integration for the TAMEX v2 REST API
Requires
- php: ^7.4|^8.0
Requires (Dev)
- phpunit/phpunit: ^9.0
README
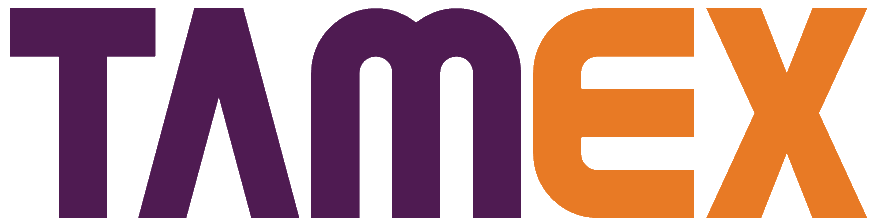
TAMEX PHP
TAMEX REST API integration for your PHP application.
Explore the docs »
View Demo
·
Report Bug
·
Request Feature
Table of Contents
About The Project
This project is a PHP integration for the TAMEX v2 REST API.
Support
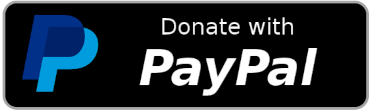
Getting Started
This package can be installed through Composer.
You can view and download TAMEX 2.7 API docs from here.
Prerequisites
Before you can start using this package, you need to contact TAMEX sales to obtain test
and live
API keys.
Installation
composer require kmuharam/tamex-php
Usage
Quick start
In the below code you find an example implementation of the three operations supported by the API.
<?php namespace MyAwesomeApp\Shipments\Services; use Kmuharam\Tamex\Requests\CreateShipmentRequest; use Kmuharam\Tamex\Requests\PrintWaybillRequest; use Kmuharam\Tamex\Requests\ShipmentStatusRequest; use Kmuharam\Tamex\Responses\CreateShipmentResponse; use Kmuharam\Tamex\Responses\PrintWaybillResponse; use Kmuharam\Tamex\Responses\ShipmentStatusResponse; class MyTamexServices { /** * @var \Kmuharam\Tamex\Services\TamexServices */ protected TamexServices $services; /** * @var boolean */ protected bool $shoudWeGoLive = false; /** * @var string */ protected string $testingApiKey = 'mytesingapikey'; /** * @var string */ protected string $liveApiKey = 'myliveapikey'; /** * Create a new instance of My Tamex Services. * * @return void */ public function __construct() { $this->shoudWeGoLive = false; // or true depending on your environment settings $this->services = new TamexServices($this->shoudWeGoLive); } /** * Create shipment request. * * @return \Kmuharam\Tamex\Responses\CreateShipmentResponse */ public function create(): CreateShipmentResponse { $createShipmentRequest = new CreateShipmentRequest(); $createShipmentRequest->apiKey = $this->shoudWeGoLive ? $this->liveApiKey : $this->testingApiKey; $createShipmentRequest->packType = '2'; // 1: Delivery, 2: Pickup $createShipmentRequest->packVendorId = 'My store name'; $createShipmentRequest->packReciverName = 'Receiver name'; $createShipmentRequest->packReciverPhone = '+966500000000'; $createShipmentRequest->packReciverCountry = 'SA'; $createShipmentRequest->packReciverCity = 'City name'; $createShipmentRequest->packReciverStreet = '26th St.'; $createShipmentRequest->packDesc = '1 item(s), weight: 3KG , price: 1200SAR.'; $createShipmentRequest->packNumPcs = 1; $createShipmentRequest->packWeight = 3; $createShipmentRequest->packCodAmount = '0'; $createShipmentRequest->packCurrencyCode = 'SAR'; $createShipmentRequest->packSenderName = 'My store name'; $createShipmentRequest->packSenderPhone = '+966500000000'; $createShipmentRequest->packSendCountry = 'SA'; $createShipmentRequest->packSendCity = 'City name'; $createShipmentRequest->packSenderStreet = '12th St., Example neighborhood, building No. 1, house No. 1'; $createShipmentRequest->packDimension = '10:10:10'; // width x height x length $response = $this->services->createShipment($createShipmentRequest); return $response; } /** * Track shipment status. * * @param string $packAWB * * @return \Kmuharam\Tamex\Responses\ShipmentStatusResponse */ public function shipmentStatus(string $packAWB): ShipmentStatusResponse { $shipmentStatusRequest = new ShipmentStatusRequest(); $shipmentStatusRequest->apiKey = $this->shoudWeGoLive ? $this->liveApiKey : $this->testingApiKey; $shipmentStatusRequest->packAWB = $packAWB; $response = $this->services->shipmentStatus($shipmentStatusRequest); return $response; } /** * Print shipment waybill. * * @param string $packAWB * * @return \Kmuharam\Tamex\Responses\PrintWaybillResponse */ public function printWaybill(string $packAWB): PrintWaybillResponse { $printWaybillRequest = new PrintWaybillRequest(); $printWaybillRequest->apiKey = $this->shoudWeGoLive ? $this->liveApiKey : $this->testingApiKey; $printWaybillRequest->packAWB = $packAWB; $response = $this->services->printWaybill($printWaybillRequest); return $response; } }
For more information about what the requests payload should look like, take a look at the below links:
- Create shipment request parameters
- Query shipment status request parameters
- Print waybill request parameters
Expected responses
Create shipment response
<?php // ... // contains original response received from the API $response->raw; // operation status code // 0 = Return tmxAWB, // 90001 = Error in Json Record Format, // 90003 = API KEY NOT AUTORIZED, // 90004 = ERROR Contact Support $response->code; // operation status text // 0 = Operation Success, // 90001 = JSON, // 90003 = Authorization, // 90004 = System $response->data; // airway bill code $response->tmxAWB; // returns true if shipment creation failed $response->hasError(); // returns true if shipment creation succeeded $response->created(); // array wrapping response properties and methods // [ // 'error' => $this->hasError(), // 'created' => $this->created(), // 'code' => $this->code, // 'data' => $this->data, // 'tmxAWB' => $this->tmxAWB, // ] $response->response(); // ...
Shipment status response
<?php // ... // contains original response received from the API $response->raw; // operation status code // 0 = Return tmxAWB, // 90001 = Error in Json Record Format, // 90003 = API KEY NOT AUTORIZED, // 90004 = ERROR Contact Support $response->code; // operation status text // 0 = Operation Success, // 90001 = JSON, // 90003 = Authorization, // 90004 = System $response->data; // airway bill code $response->awb; // status message code $response->status; // Status update date and time $response->updateOn; // status message string $response->message; // returns true if shipment creation failed $response->hasError(); // returns true if shipment exists $response->exists(); // array wrapping response properties and methods // [ // 'error' => $this->hasError(), // 'exists' => $this->exists(), // 'code' => $this->code, // 'data' => $this->data, // 'awb' => $this->awb, // 'status' => $this->status, // 'updateOn' => $this->updateOn, // 'message' => $this->message, // ] $response->response(); // ...
Print waybill response
<?php // ... // contains original response received from the API $response->raw; // operation status code // 0 = Return tmxAWB, // 90001 = Error in Json Record Format, // 90003 = API KEY NOT AUTORIZED, // 90004 = ERROR Contact Support $response->code; // operation status text // 0 = Operation Success, // 90001 = JSON, // 90003 = Authorization, // 90004 = System $response->data; // waybill pdf as base64 string $response->contents; // returns true if shipment creation failed $response->hasError(); // returns true if shipment exists $response->exists(); // array wrapping response properties and methods // [ // 'error' => $this->hasError(), // 'exists' => $this->exists(), // 'code' => $this->code, // 'data' => $this->data, // 'contents' => $this->contents, // ] $response->response(); // ...
Roadmap
- Add support for webhooks
- Add documentation pages at muharam.dev
See the open issues for a full list of proposed features (and known issues).
Contributing
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are greatly appreciated.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the tag "enhancement". Don't forget to give the project a star! Thanks again!
- Fork the Project
- Create your Feature Branch (
git checkout -b feature/AmazingFeature
) - Commit your Changes (
git commit -m 'Add some AmazingFeature'
) - Push to the Branch (
git push origin feature/AmazingFeature
) - Open a Pull Request
License
Distributed under the MIT License. See LICENSE.md
for more information.
Contact
Khalid Muharam - devel@muharam.dev
Project Link: https://github.com/kmuharam/tamex-php